Mongock with spring boot 3
Recently I wanted to add mongock to a spring boot 3 project and I was surprised to see the official docs are not yet updated with the actual requirements for the installation so I spent good 2 hours trying to figure out just how to make it work.
In this post I want to share the steps I took so it will hopefully help someone else or my future self.
- Add the necessary dependencies
<dependencyManagement>
<dependencies>
<dependency>
<groupId>io.mongock</groupId>
<artifactId>mongock-bom</artifactId>
<version>5.5.0</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
... dependencies
<dependency>
<groupId>io.mongock</groupId>
<artifactId>mongock-springboot-v3</artifactId>
</dependency>
<dependency>
<groupId>io.mongock</groupId>
<artifactId>mongodb-springdata-v4-driver</artifactId>
</dependency>
- Add the configuration
@Configuration
public class MongockConfig {
private final MongoTemplate mongoTemplate;
public MongockConfig(MongoTemplate mongoTemplate) {
this.mongoTemplate = mongoTemplate;
}
@Bean
public SpringDataMongoV4Driver springDataMongoV3Driver() {
return SpringDataMongoV4Driver.withDefaultLock(mongoTemplate);
}
@Bean
public MongockApplicationRunner mongockApplicationRunner(SpringDataMongoV4Driver driver, ApplicationContext springContext) {
return MongockSpringboot.builder()
.setDriver(driver)
.addMigrationScanPackages(List.of("com.example.backend.common.database.migration"))
.setSpringContext(springContext)
.buildApplicationRunner();
}
}
- Write your change units
@ChangeUnit(id = "productTextSearchIndex", order = "001", author = "Nermin")
public class ProductTextSearchIndex {
private final MongoTemplate mongoTemplate;
public ProductTextSearchIndex(MongoTemplate mongoTemplate) {
this.mongoTemplate = mongoTemplate;
}
@Execution
public void changeset() {
mongoTemplate.indexOps("product")
.ensureIndex(new TextIndexDefinition.TextIndexDefinitionBuilder()
.onField("title")
.onField("description")
.onField("tags")
.build());
}
@RollbackExecution
public void rollback() {
mongoTemplate.indexOps("product").dropIndex("title_text_description_text_tags_text");
}
}
That's it, happy coding 😄
Here's some links of the official docs and additional resources:
Mongock Documentation
Mongock Documentation
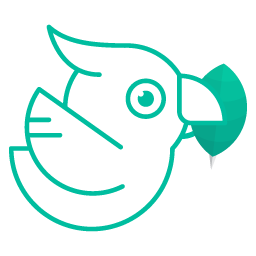
JHLite: Spring Boot 3 - upgrade Mongock · Issue #4067 · jhipster/jhipster-lite
Links: #3601 #3832 You can use this branch: https://github.com/jhipster/jhipster-lite/tree/spring-boot-3 and submit a pull request against this branch Related to this ticket mongock/mongock#583 So…
Member discussion