Exploring tanstack-start [1]—install tailwind and mantine
In the previous post we've setup the basic structure of a tanstack start project. In case you want to revisit that:
https://scriptkiddy.pro/exploring-tanstack-start-next-best-full-stack-framework/
Today we're going to add Mantine, the UI library, and tailwind.
First, install mantine packages:
npm install @mantine/core @mantine/hooks @mantine/form @mantine/dates dayjs @mantine/charts recharts@2 @mantine/notifications @mantine/code-highlight @mantine/tiptap @tiptap/pm @tiptap/react @tiptap/extension-link @tiptap/starter-kit @mantine/dropzone @mantine/carousel embla-carousel-react@^7.1.0 @mantine/spotlight @mantine/modals @mantine/nprogress
Install PostCSS plugins and postcss-preset-mantine:
npm install --save-dev postcss postcss-preset-mantine postcss-simple-vars
Add postcss.config.cjs to the root directory
// postcss.config.cjs
module.exports = {
plugins: {
'postcss-preset-mantine': {},
'postcss-simple-vars': {
variables: {
'mantine-breakpoint-xs': '36em',
'mantine-breakpoint-sm': '48em',
'mantine-breakpoint-md': '62em',
'mantine-breakpoint-lg': '75em',
'mantine-breakpoint-xl': '88em',
},
},
},
};
Import mantine styles
// app/styles/app.css
@import '@mantine/core/styles.css';
Update __root route
import {
Outlet,
ScrollRestoration,
createRootRoute,
createRootRouteWithContext,
} from '@tanstack/react-router'
import type { QueryClient } from "@tanstack/react-query";
import appCss from '../styles/app.css?url'
import { createTheme, MantineProvider } from '@mantine/core';
import { Meta, Scripts } from '@tanstack/start'
import type { ReactNode } from 'react'
export const Route = createRootRouteWithContext<{
queryClient: QueryClient
}>()({
beforeLoad: async () => {
// Get user data
},
head: () => ({
meta: [
{
charSet: 'utf-8',
},
{
name: 'viewport',
content: 'width=device-width, initial-scale=1',
},
{
title: 'TanStack Start Starter',
},
],
links: [{rel: "stylesheet", href: appCss}]
}),
component: RootComponent,
})
function RootComponent() {
return (
<MantineProvider>
<RootDocument>
<Outlet />
</RootDocument>
</MantineProvider>
)
}
function RootDocument({ children }: Readonly<{ children: ReactNode }>) {
return (
<html>
<head>
<Meta />
</head>
<body>
{children}
<ScrollRestoration />
<Scripts />
{children}
<ScrollRestoration />
<Scripts />
</body>
</html>
)
Adding tailwind to tanstack-start
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init
Add Tailwind to your PostCSS configuration
// postcss.config.cjs
module.exports = {
plugins: {
'postcss-preset-mantine': {},
'postcss-simple-vars': {
variables: {
'mantine-breakpoint-xs': '36em',
'mantine-breakpoint-sm': '48em',
'mantine-breakpoint-md': '62em',
'mantine-breakpoint-lg': '75em',
'mantine-breakpoint-xl': '88em',
},
},
tailwindcss: {},
autoprefixer: {},
},
};
Configure your template paths
/** @type {import('tailwindcss').Config} */
export default {
content: ["./app/**/*.{html,js,jsx,ts,tsx}"],
theme: {
extend: {},
},
plugins: [],
}
Add the Tailwind directives to your CSS
// app/styles/app.css
@import '@mantine/core/styles.css';
@tailwind base;
@tailwind components;
@tailwind utilities;
Now let's see if this works, Modify the home page route
// app/routes/index.tsx
import { createFileRoute, useRouter } from '@tanstack/react-router'
import { Button } from '@mantine/core'
export const Route = createFileRoute('/')({
component: Home,
loader: async () => await Promise.resolve(1),
})
function Home() {
const router = useRouter()
const state = Route.useLoaderData()
return (
<div className='max-w-screen-lg w-full mx-auto'>
<h1 className='text-4xl font-bold text-center mt-8'>Hello, world!</h1>
</div>
)
}
You should see a page like this:
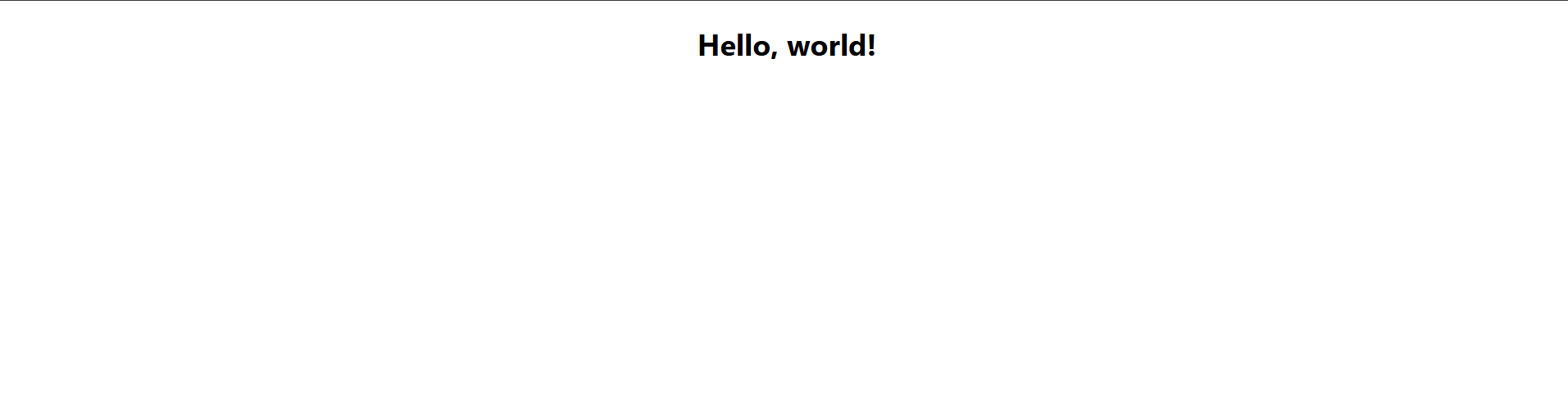
Member discussion